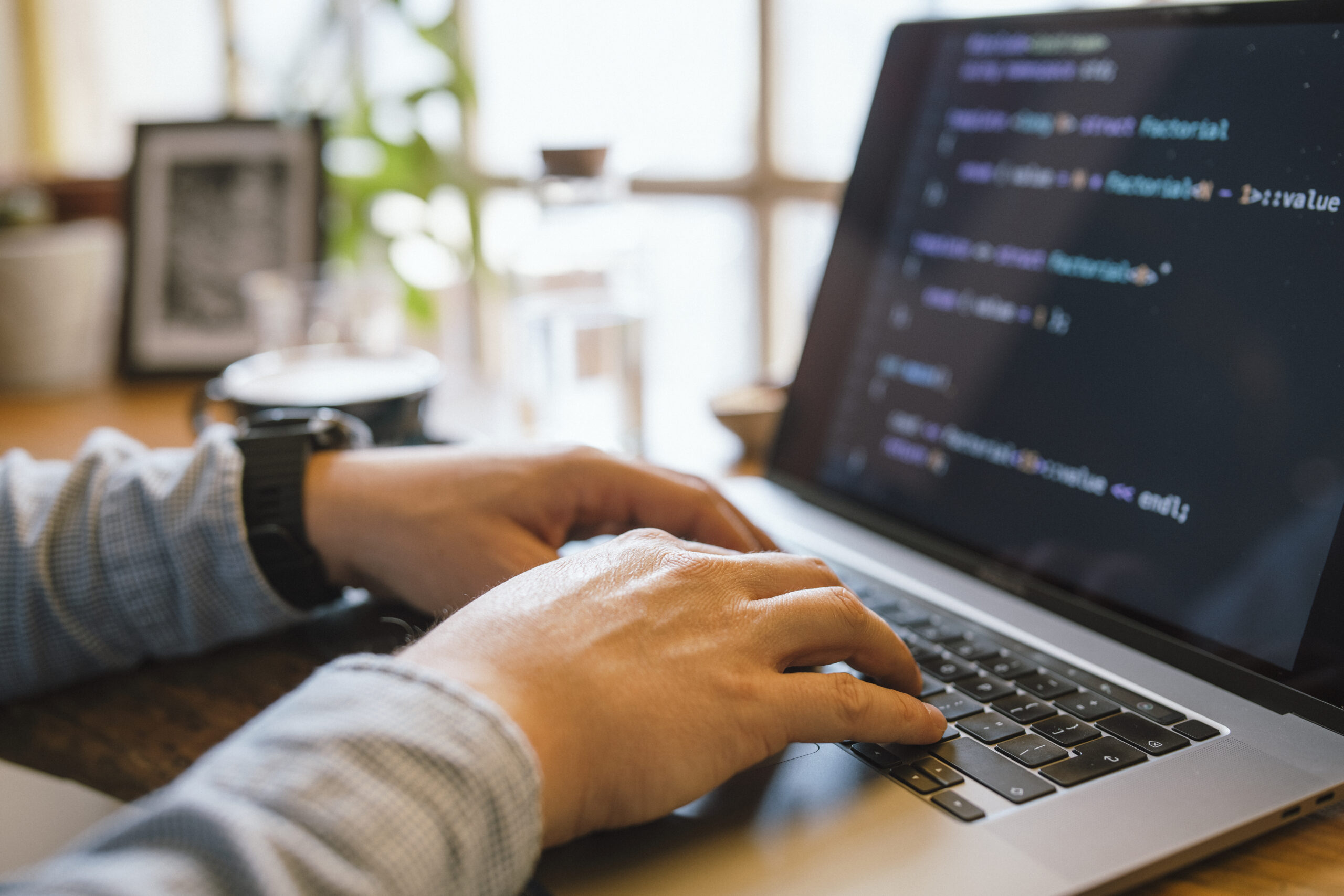
Debugging is Just about the most necessary — nonetheless often disregarded — capabilities in a very developer’s toolkit. It's not just about repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to resolve challenges successfully. Irrespective of whether you are a starter or simply a seasoned developer, sharpening your debugging abilities can preserve several hours of frustration and drastically boost your productivity. Listed below are numerous techniques that can help builders amount up their debugging sport by me, Gustavo Woltmann.
Master Your Resources
One of many quickest techniques developers can elevate their debugging skills is by mastering the instruments they use every day. While creating code is a single Portion of enhancement, recognizing ways to connect with it properly for the duration of execution is equally vital. Fashionable development environments occur Geared up with effective debugging capabilities — but several developers only scratch the surface of what these instruments can do.
Consider, for example, an Built-in Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code on the fly. When utilised properly, they Permit you to notice specifically how your code behaves all through execution, that's invaluable for monitoring down elusive bugs.
Browser developer equipment, like Chrome DevTools, are indispensable for front-end developers. They allow you to inspect the DOM, watch community requests, view serious-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can change frustrating UI difficulties into manageable responsibilities.
For backend or program-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Regulate over managing procedures and memory management. Mastering these tools might have a steeper Understanding curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn into snug with Edition Manage units like Git to know code historical past, come across the precise instant bugs were being launched, and isolate problematic improvements.
Finally, mastering your tools implies heading outside of default settings and shortcuts — it’s about building an personal expertise in your enhancement environment to ensure that when concerns crop up, you’re not shed in the dark. The better you realize your resources, the more time you'll be able to shell out fixing the actual dilemma in lieu of fumbling by the method.
Reproduce the challenge
The most essential — and sometimes disregarded — methods in powerful debugging is reproducing the trouble. In advance of leaping to the code or building guesses, builders need to have to make a steady natural environment or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug gets to be a game of opportunity, often resulting in wasted time and fragile code changes.
The initial step in reproducing a difficulty is accumulating just as much context as you can. Inquire thoughts like: What actions led to The problem? Which atmosphere was it in — advancement, staging, or output? Are there any logs, screenshots, or error messages? The more depth you've, the a lot easier it turns into to isolate the precise disorders beneath which the bug takes place.
As soon as you’ve collected plenty of info, seek to recreate the condition in your local ecosystem. This might mean inputting the exact same information, simulating equivalent consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the sting circumstances or point out transitions involved. These exams not simply assist expose the challenge but will also protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it might transpire only on certain working devices, browsers, or less than specific configurations. Employing equipment like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the issue isn’t only a action — it’s a mentality. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be already halfway to repairing it. That has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination opportunity fixes properly, and connect more Evidently with all your workforce or people. It turns an summary criticism right into a concrete problem — Which’s the place developers thrive.
Read and Understand the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then observing them as frustrating interruptions, builders really should study to deal with error messages as immediate communications with the technique. They generally inform you just what happened, where by it took place, and often even why it transpired — if you understand how to interpret them.
Get started by looking through the message carefully As well as in total. Many builders, especially when below time pressure, look at the initial line and immediately start out producing assumptions. But further while in the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it point to a certain file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the dependable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable designs, and Mastering to acknowledge these can drastically accelerate your debugging course of action.
Some errors are obscure or generic, As well as in These scenarios, it’s vital to look at the context wherein the error transpired. Test related log entries, enter values, and recent alterations inside the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial issues and provide hints about likely bugs.
In the long run, error messages are usually not your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent equipment in the developer’s debugging toolkit. When applied proficiently, it provides actual-time insights into how an application behaves, assisting you realize what’s taking place beneath the hood without needing to pause execution or step through the code line by line.
A fantastic logging system starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, Mistake, and Deadly. Use DEBUG for thorough diagnostic data in the course of advancement, Information for common occasions (like successful begin-ups), WARN for potential challenges that don’t crack the appliance, ERROR for precise problems, and Lethal when the system can’t go on.
Prevent flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure critical messages and slow down your procedure. Focus on critical functions, state improvements, input/output values, and important final decision points in the code.
Structure your log messages Obviously and consistently. Include things like context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular precious in manufacturing environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, clever logging is about equilibrium and clarity. Using a perfectly-believed-out logging technique, you can decrease the time it's going to take to spot concerns, gain deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a technical activity—it is a sort of investigation. To effectively determine and correct bugs, builders will have to approach the process just like a detective resolving a secret. This state of mind will help stop working elaborate problems into workable sections and abide by clues logically to uncover the root lead to.
Start out by accumulating proof. Think about the signs or symptoms of the problem: mistake messages, incorrect output, or performance problems. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and user reports to piece together a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What could possibly be creating this habits? Have any adjustments not too long ago been produced to the codebase? Has this issue transpired prior to under similar instances? The goal should be to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge within a managed natural environment. For those who suspect a certain function or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, request your code questions and Permit the outcomes lead you nearer to the truth.
Pay back near attention to compact specifics. Bugs often cover within the the very least predicted locations—similar to a missing semicolon, an off-by-a person error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out absolutely comprehension it. Temporary fixes may possibly hide the true trouble, only for it to resurface later on.
Lastly, hold notes on what you tried using and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming concerns and enable Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated programs.
Generate Tests
Creating checks is among the most effective approaches to increase your debugging competencies and Over-all development efficiency. Tests not just support capture bugs early but also serve as a safety net that gives you self-confidence when producing alterations on your codebase. A perfectly-analyzed software is much easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These little, isolated assessments can speedily reveal no matter whether a particular piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, considerably reducing some time expended debugging. Device exams are In particular handy for catching regression bugs—troubles that reappear right after previously being preset.
Next, combine integration exams and finish-to-end assessments into your workflow. These support make certain that various aspects of your application function alongside one another efficiently. They’re specially beneficial for catching bugs that occur in elaborate programs with numerous factors or providers interacting. If some thing breaks, your assessments can let you know which Element of the pipeline failed and under what disorders.
Composing tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging an issue, producing a failing test that reproduces the bug might be a robust first step. When the exam fails continually, you are able to center on correcting the bug and observe your take a look at go when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In get more info short, creating tests turns debugging from a annoying guessing video game right into a structured and predictable procedure—supporting you capture extra bugs, faster and even more reliably.
Just take Breaks
When debugging a tough issue, it’s simple to be immersed in the problem—looking at your display for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks will help you reset your mind, decrease aggravation, and often see the issue from a new standpoint.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind gets considerably less productive at difficulty-solving. A short wander, a espresso split, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the history.
Breaks also support avoid burnout, Particularly during extended debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you in advance of.
In the event you’re trapped, an excellent general guideline is usually to set a timer—debug actively for 45–sixty minutes, then take a five–ten minute split. Use that point to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more effective debugging Eventually.
In short, getting breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your development. Debugging is a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is a lot more than simply a temporary setback—It really is a chance to mature as a developer. No matter whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, every one can instruct you something useful should you make time to replicate and review what went wrong.
Begin by asking by yourself some vital questions once the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or being familiar with and assist you Establish much better coding patterns going forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you could proactively stay away from.
In group environments, sharing Whatever you've discovered from a bug with all your friends could be Specifically potent. Whether it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Some others stay away from the exact same difficulty boosts crew effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In any case, some of the finest developers are certainly not the ones who produce ideal code, but those that repeatedly discover from their issues.
Ultimately, Each individual bug you correct provides a fresh layer towards your ability established. So next time you squash a bug, take a instant to reflect—you’ll appear absent a smarter, much more able developer thanks to it.
Conclusion
Increasing your debugging expertise can take time, practice, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a possibility to be better at Everything you do.