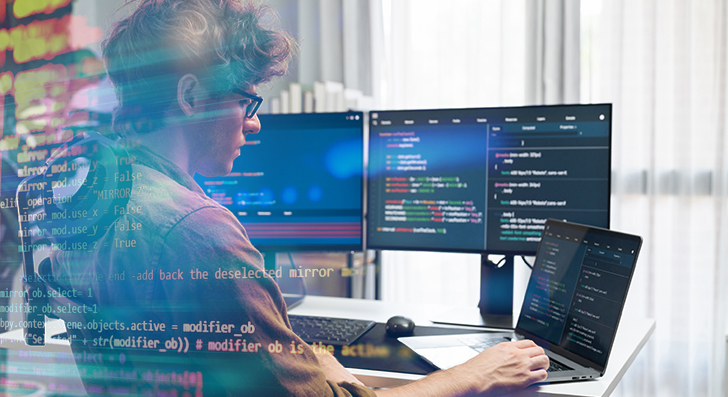
Scalability implies your software can take care of progress—much more users, additional knowledge, and a lot more site visitors—with out breaking. Like a developer, developing with scalability in your mind saves time and worry later on. Right here’s a transparent and useful guide that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's strategy from the start. Numerous apps fail if they develop speedy due to the fact the first design and style can’t deal with the additional load. As a developer, you'll want to think early about how your procedure will behave under pressure.
Start off by designing your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. Rather, use modular layout or microservices. These styles break your app into lesser, independent elements. Each individual module or services can scale on its own with no influencing the whole program.
Also, think of your database from day one particular. Will it will need to take care of a million consumers or just 100? Pick the ideal type—relational or NoSQL—determined by how your details will grow. Program for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential place is to stay away from hardcoding assumptions. Don’t write code that only functions below existing problems. Give thought to what would occur Should your consumer foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure patterns that assistance scaling, like message queues or event-driven systems. These support your app manage a lot more requests without having acquiring overloaded.
Any time you Make with scalability in mind, you're not just preparing for achievement—you happen to be lowering potential head aches. A effectively-planned method is easier to take care of, adapt, and increase. It’s greater to get ready early than to rebuild later on.
Use the proper Databases
Picking out the proper database is often a essential Portion of building scalable applications. Not all databases are built the exact same, and using the Incorrect you can sluggish you down or even bring about failures as your app grows.
Get started by knowledge your facts. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to deal with extra site visitors and details.
When your data is much more flexible—like consumer exercise logs, solution catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing large volumes of unstructured or semi-structured knowledge and will scale horizontally far more easily.
Also, contemplate your browse and create designs. Are you presently carrying out numerous reads with much less writes? Use caching and read replicas. Do you think you're handling a large produce load? Look into databases that will take care of high compose throughput, or maybe party-based info storage programs like Apache Kafka (for short-term knowledge streams).
It’s also clever to Imagine ahead. You may not require Superior scaling characteristics now, but deciding on a databases that supports them usually means you received’t want to change later on.
Use indexing to hurry up queries. Prevent unwanted joins. Normalize or denormalize your details depending on your access styles. And always monitor database efficiency while you expand.
In a nutshell, the correct database is determined by your app’s structure, speed needs, And exactly how you hope it to develop. Consider time to pick sensibly—it’ll help you save many hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual small hold off provides up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by crafting cleanse, basic code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Resolution if a simple a person will work. Keep your functions shorter, centered, and easy to check. Use profiling equipment to locate bottlenecks—sites the place your code requires much too extended to operate or employs excessive memory.
Subsequent, evaluate your database queries. These normally sluggish issues down much more than the code itself. Be certain Each and every question only asks for the data you really need to have. Avoid Decide on *, which fetches everything, and alternatively find certain fields. Use indexes to hurry up lookups. And steer clear of undertaking a lot of joins, Specifically throughout large tables.
Should you notice precisely the same details becoming asked for many times, use caching. Shop the outcome quickly using resources like Redis or Memcached therefore you don’t have to repeat costly functions.
Also, batch your databases operations whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to exam with large datasets. Code and queries that function wonderful with a hundred records may crash after they have to manage one million.
In a nutshell, scalable applications are rapidly applications. Keep the code limited, your queries lean, and use caching when wanted. These ways help your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your app grows, it's to deal with a lot more consumers and a lot more targeted traffic. If almost everything goes by just one server, it can quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these equipment support maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As opposed to a single server undertaking every one of the perform, the load balancer routes customers to different servers dependant on availability. This suggests no solitary server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this very easy to create.
Caching is about storing data quickly so it may be reused quickly. When users ask for exactly the same information yet again—like a product web site or maybe a profile—you don’t must fetch it within the databases each and every time. You can provide it in the cache.
There's two frequent different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) suppliers static data files close to the consumer.
Caching reduces databases load, increases speed, and would make your app additional efficient.
Use caching for things which don’t change usually. And normally ensure your cache is current when information does transform.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they help your application handle far more buyers, stay quickly, and Get better from problems. If you plan to increase, you need both equally.
Use Cloud and Container Tools
To construct scalable apps, you require tools that allow your application improve conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors improves, you can add much more sources with just a few clicks or immediately utilizing car-scaling. here When targeted traffic drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app instead of managing infrastructure.
Containers are A further critical Resource. A container deals your app and everything it must operate—code, libraries, configurations—into one particular unit. This makes it uncomplicated to maneuver your app between environments, from the laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs numerous containers, tools like Kubernetes help you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it quickly.
Containers also ensure it is easy to different elements of your application into companies. You are able to update or scale sections independently, which can be perfect for efficiency and reliability.
In a nutshell, making use of cloud and container applications signifies you can scale rapidly, deploy effortlessly, and Get well quickly when troubles occur. In order for you your app to expand without the need of limitations, start out utilizing these instruments early. They save time, minimize hazard, and assist you to keep focused on constructing, not correcting.
Keep track of Almost everything
If you don’t keep track of your software, you received’t know when things go Improper. Checking can help the thing is how your app is executing, place challenges early, and make better choices as your app grows. It’s a essential Element of developing scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it takes for customers to load pages, how often errors take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Put in place alerts for critical troubles. One example is, If the reaction time goes previously mentioned a limit or perhaps a service goes down, you should get notified immediately. This allows you take care of difficulties rapidly, typically just before customers even notice.
Monitoring is also practical any time you make alterations. Should you deploy a brand new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it triggers real problems.
As your app grows, traffic and facts enhance. With out checking, you’ll overlook indications of difficulties till it’s much too late. But with the best resources in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your program and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big firms. Even small apps have to have a powerful Basis. By designing meticulously, optimizing wisely, and using the right equipment, you can Construct applications that develop efficiently without the need of breaking under pressure. Start off small, Feel major, and build wise.